Tic-Tac-Toe is a classic game that has entertained people for generations. In this article, we’ll explore how to create a professional-grade Tic-Tac-Toe game using HTML, CSS, and JavaScript.

By the end of this tutorial, you’ll have a fully functional game that you can proudly showcase on your website.
Step 1: Setting Up the HTML Structure
First, let’s create the HTML structure for our tic tac toe game board. Lets start from a basic html structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Tic Tac Toe</title>
<link rel="stylesheet" type="text/css" href="stylesheet.css">
</head>
<body>
First we add banner sports inside body tag:
<div class="banner top-banner">Place Your Top Banner Code Here</div>
<div class="banner left-banner">Place Your Left Banner Code Here</div>
<div class="banner right-banner">Place Your Right Banner Code Here</div>
Now I added a container with options and cell for tic tac toe game and close the body and html tag.
<div class="container">
<br><br><br><br><h1>Tic Tac Toe</h1>
<div class="options">
<label for="mode">Select Mode:</label>
<select name="mode" id="mode">
<option value="multiplayer">Multiplayer</option>
<option value="singleplayer">Single Player</option>
</select>
</div>
<div class="game-board">
<div class="cell" id="0"></div>
<div class="cell" id="1"></div>
<div class="cell" id="2"></div>
<div class="cell" id="3"></div>
<div class="cell" id="4"></div>
<div class="cell" id="5"></div>
<div class="cell" id="6"></div>
<div class="cell" id="7"></div>
<div class="cell" id="8"></div>
</div>
<div class="status"></div>
<button class="reset-btn">Reset</button>
</div>
<script type="text/javascript" src="javascript.js"></script>
</body>
</html>
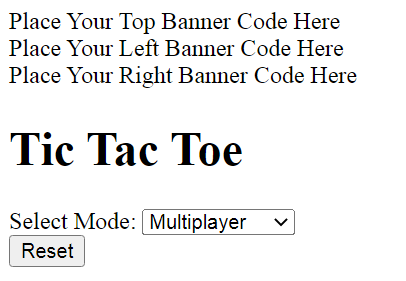
Step 2: Styling the Game with CSS
Next, let’s style our game board to make it visually appealing and intuitive for users.
body {
background: linear-gradient(to bottom right, #4facfe, #00f2fe);
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
padding: 20px;
position: relative; /* Ensure position relative for absolute positioning */
}
Let’s add some styling to the banners to adjust them on designated spots.
.banner {
position: absolute;
width: 100%; /* Occupy full width */
text-align: center;
line-height: 50px;
}
.top-banner {
top: 0;
}
.left-banner {
top: 120px; /* Height of top banner */
left: 0;
width: 200px;
height: calc(100% - 120px); /* Adjust height to fill remaining space */
}
.right-banner {
top: 120px; /* Height of top banner */
right: 0;
width: 200px;
height: calc(100% - 120px); /* Adjust height to fill remaining space */
}
Add styling to the Game section:
.container {
text-align: center;
}
.game-board {
display: grid;
grid-template-columns: repeat(3, 100px);
grid-gap: 10px;
margin: 20px auto;
}
.cell {
width: 100px;
height: 100px;
background: #fff;
border: 2px solid #000;
display: flex;
justify-content: center;
align-items: center;
font-size: 2em;
cursor: pointer;
}
.status {
margin-bottom: 10px;
font-size: 1.5em;
}
.reset-btn {
padding: 10px 20px;
font-size: 1em;
background: #f44336;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
.options {
margin-top: 20px;
}
.options label {
font-size: 1.2em;
}
.options select {
padding: 5px;
font-size: 1em;
}
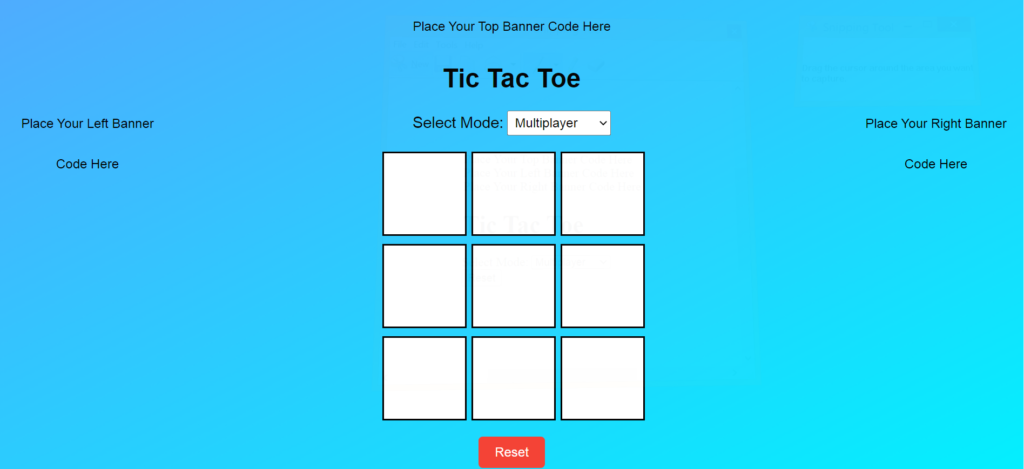
Step 3: Implementing the Game Logic with JavaScript
Now, let’s add the JavaScript code to handle the game logic. We’ll implement functions to handle player moves, check for a winner, and reset the game.
document.addEventListener('DOMContentLoaded', function () {
const cells = document.querySelectorAll('.cell');
const status = document.querySelector('.status');
const resetButton = document.querySelector('.reset-btn');
const modeSelect = document.querySelector('#mode');
let currentPlayer = 'X';
let gameActive = true;
let player1Wins = 0;
let player2Wins = 0;
let draws = 0;
const winningCombinations = [
[0, 1, 2], [3, 4, 5], [6, 7, 8],
[0, 3, 6], [1, 4, 7], [2, 5, 8],
[0, 4, 8], [2, 4, 6]
];
function handleCellClick(event) {
const cell = event.target;
const cellIndex = parseInt(cell.id);
if (cell.textContent || !gameActive) return;
cell.textContent = currentPlayer;
if (checkWin()) {
if (currentPlayer === 'X') {
player1Wins++;
} else {
player2Wins++;
}
updateStatistics();
endGame(`${currentPlayer} wins!`);
return;
}
if (checkDraw()) {
draws++;
updateStatistics();
endGame('It\'s a draw!');
return;
}
currentPlayer = currentPlayer === 'X' ? 'O' : 'X';
status.textContent = `Player ${currentPlayer}'s turn`;
if (modeSelect.value === 'singleplayer' && currentPlayer === 'O') {
// If it's the system's turn in single-player mode, make a move
makeSystemMove();
}
}
function makeSystemMove() {
// Simple AI: System makes a random move
const emptyCells = [...cells].filter(cell => cell.textContent === '');
const randomIndex = Math.floor(Math.random() * emptyCells.length);
emptyCells[randomIndex].textContent = currentPlayer;
if (checkWin()) {
player2Wins++;
updateStatistics();
endGame('System wins!');
} else if (checkDraw()) {
draws++;
updateStatistics();
endGame('It\'s a draw!');
} else {
currentPlayer = 'X';
status.textContent = `Player ${currentPlayer}'s turn`;
}
}
function checkWin() {
return winningCombinations.some(combination => {
return combination.every(index => {
return cells[index].textContent === currentPlayer;
});
});
}
function checkDraw() {
return [...cells].every(cell => {
return cell.textContent !== '';
});
}
function endGame(message) {
gameActive = false;
status.textContent = message;
}
function resetGame() {
cells.forEach(cell => {
cell.textContent = '';
});
currentPlayer = 'X';
gameActive = true;
status.textContent = `Player ${currentPlayer}'s turn`;
if (modeSelect.value === 'singleplayer' && currentPlayer === 'O') {
// If it's the system's turn at the start of the game, make a move
makeSystemMove();
}
}
function updateStatistics() {
if (modeSelect.value === 'multiplayer') {
status.textContent = `Player 1: ${player1Wins}, Draw: ${draws}, Player 2: ${player2Wins}`;
} else {
status.textContent = `Player: ${player1Wins}, Draw: ${draws}, System: ${player2Wins}`;
}
}
cells.forEach(cell => {
cell.addEventListener('click', handleCellClick);
});
resetButton.addEventListener('click', resetGame);
modeSelect.addEventListener('change', function () {
resetGame();
player1Wins = 0;
player2Wins = 0;
draws = 0;
updateStatistics();
});
updateStatistics();
});
Download Source Code
To download the complete source code Click on the link below. Beaware the code is password protected, so to access the code please enter this password:
q7tOOXV89f
Step 4: Conclusion
Congratulations! You've successfully built a professional Tic-Tac-Toe game using HTML, CSS, and JavaScript. Your game is now fully functional and ready to be played. Feel free to customize the styling and add additional features to make it your own.
In this tutorial, you've learned how to:
- Create the HTML structure for the game board
- Style the game using CSS to enhance the user experience
- Implement the game logic with JavaScript to handle player moves, check for a winner, and reset the game
Now, go ahead and showcase your Tic-Tac-Toe game on your website or portfolio. Happy gaming!